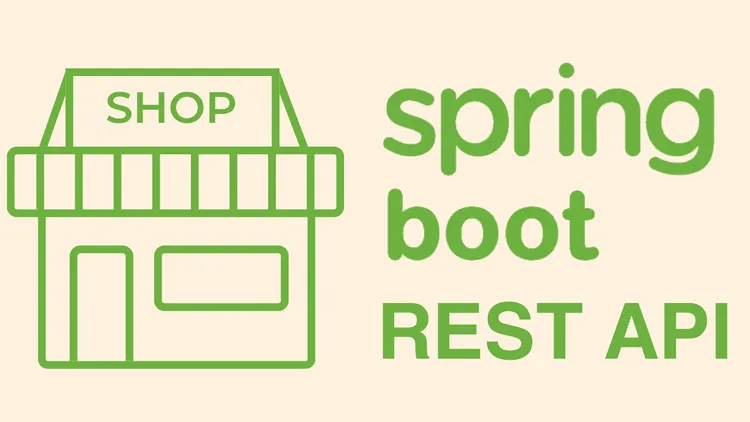
Java API Project
The project focuses on the development of an API for a store, with the aim of managing products, sales, and customers. This system will allow the store to efficiently manage its sales operations and product inventory, facilitating the registration and handling of these processes through a web interface and a mobile application in the future.
Check out the project repository
The proposed solution is structured following the multi-layer model (also known as layered architecture), a design pattern widely used in enterprise applications, especially with the Spring Boot framework in the Java ecosystem.
Check out -> A standard multi-layer structure commonly used in Spring Boot
Below is a detailed explanation of how this model applies to the project:
1. Presentation Layer (Controller)
In the context of this API, the presentation layer is responsible for interacting with the end-users (the store owner and her employees) through two types of HTTP clients: a web application and, potentially, a mobile application. Using Spring Boot, this layer is composed mainly of controllers (annotated with @RestController
), which listen to HTTP requests (GET, POST, DELETE, PUT) and respond with the requested data or the result of an operation. The controllers make calls to the service layer to execute the business logic.
2. Service Layer (Service)
The service layer, implemented with classes annotated with @Service
, contains the application鈥檚 business logic. Here, methods are defined for operations such as CRUD of products, clients, and sales; querying products with low availability; obtaining details of specific sales; and the aggregation of sales data. This layer acts as an intermediary between the presentation layer and the data access layer, ensuring that the requested operations are executed according to established business rules.
3. Data Access Layer (Repository)
This layer interacts directly with the data source (for example, a relational database) to perform CRUD operations on the application entities: Product, Client, and Sale. In Spring Boot, this is achieved through the use of repositories (annotated with @Repository
), which extend interfaces like JpaRepository
to provide ready-to-use data access methods, as well as the ability to define custom queries. This layer abstracts the complexity of direct database management from the other layers of the application.
4. Model Layer (Model)
Finally, the model layer defines the business entities (Product, Sale, Client) with their respective properties and relationships. Using JPA (Java Persistence API) and annotations such as @Entity
, Spring Boot maps these classes to database tables, facilitating the management of data persistence and relationships between entities.
The project applies the multi-layer model, separating responsibilities into different layers that interact with each other to facilitate the development, maintenance, and scalability of the application. Spring Boot significantly simplifies the implementation of each layer through the use of annotations and dependency injection, allowing developers to focus on business logic and providing value to end-users. This approach ensures that the API can be consumed by both web and mobile applications, meeting the requirements of the store owner and adapting to future expansions or modifications.
The provided image showcases the package structure for the aforementioned store. This structure mirrors the layered architecture and the organization of responsibilities within the code.
The application is divided into several packages that group together classes and interfaces with similar responsibilities:
Packages and Their Role in the Multi-Layer Architecture
-
com.marianadwarka.tienda.controller
- Contains controller classes (
ClienteController
,ProductoController
,VentaController
) which act as part of the presentation layer. These classes handle incoming HTTP requests, invoke operations from the service layer, and return the appropriate responses to the client.
- Contains controller classes (
-
com.marianadwarka.tienda.dto
- Defines the data transfer objects (DTOs), such as
MayorVentaDTO
andProductosPorVentaDTO
. DTOs are used to transfer specific information between sublayers, particularly from the business logic to the presentation layer, facilitating data exchange and reducing direct exposure of the model entities.
- Defines the data transfer objects (DTOs), such as
-
com.marianadwarka.tienda.model
- Contains the domain entities (
Cliente
,Producto
,Venta
) that represent the model layer. These classes are typically annotated with@Entity
and reflect the structure of the database in the code.
- Contains the domain entities (
-
com.marianadwarka.tienda.repository
- Houses interfaces for the data access layer, like
IClienteRepository
,IProductoRepository
,IVentaRepository
. These interfaces extend frameworks such as JPA, enabling an abstraction of the database access.
- Houses interfaces for the data access layer, like
-
com.marianadwarka.tienda.service
- Includes interfaces (
IClienteService
,IProductoService
,IVentaService
) and their implementations (ClienteService
,ProductoService
,VentaService
). The interfaces define the business logic and the operations that can be carried out, while the implementations concrete these definitions.
- Includes interfaces (
Use of Interfaces for Service and Repository Layers
As observed, the implementation of layers through interfaces is a recommended practice with multiple advantages. For example, interfaces help to decouple the application鈥檚 layers. The presentation layer does not need to know how operations are implemented in the service layer; it only needs to know the interface. This facilitates changes and the maintainability of the code.
Additionally, interfaces allow for multiple implementations. This is useful if the business logic needs to vary according to different contexts, without changing how the clients of the service layer interact with it.
Another important aspect is that interfaces act as contracts that define what operations are available, which aids in the clarity of the design and the expectation of what each part of the system does.
The developed API represents a technical solution for the operational needs of an expanding store. Leveraging the strengths of Spring Boot and the multi-layer architecture, a decoupled system that is easy to test, maintain, and expand has been achieved. The organization of the code into well-defined packages, along with the implementation of interfaces for the service and data access layers, ensures the ability to adapt the application to future requirements with minimal effort.
Espa帽ol
El proyecto se enfoca en el desarrollo de una API para una tienda, con el objetivo de gestionar productos, ventas y clientes. Este sistema permitir谩 a la tienda administrar de manera eficiente sus operaciones de venta y el stock de sus productos, facilitando el registro y manejo de estos procesos a trav茅s de una interfaz web y una aplicaci贸n m贸vil en el futuro.
Revisar repositorio del proyecto
La soluci贸n propuesta se estructura siguiendo el modelo multicapas (tambi茅n conocido como arquitectura en capas), un patr贸n de dise帽o ampliamente utilizado en aplicaciones empresariales, especialmente con el framework Spring Boot en el ecosistema de Java.
Revisar -> A standard multi-layer structure commonly used in Spring Boot
A continuaci贸n, se detalla c贸mo se aplica este modelo al proyecto:
1. Capa de presentaci贸n (Controller)
En el contexto de esta API, la capa de presentaci贸n se encarga de interactuar con los usuarios finales (la due帽a de la tienda y sus empleados) a trav茅s de dos tipos de clientes HTTP: una aplicaci贸n web y, potencialmente, una aplicaci贸n m贸vil. Utilizando Spring Boot, esta capa est谩 compuesta principalmente por controladores (anotados con @RestController
), que escuchan las solicitudes HTTP (GET, POST, DELETE, PUT) y responden con los datos solicitados o el resultado de una operaci贸n. Los controladores hacen llamadas a la capa de servicio para ejecutar la l贸gica de negocio.
2. Capa de Servicio (Service)
La capa de servicio, implementada con clases anotadas con @Service
, contiene la l贸gica de negocio de la aplicaci贸n. Aqu铆 se definen m茅todos para operaciones como el CRUD de productos, clientes, y ventas; la consulta de productos con baja disponibilidad; la obtenci贸n de detalles de ventas espec铆ficas; y la agregaci贸n de datos de ventas. Esta capa act煤a como un intermediario entre la capa de presentaci贸n y la capa de acceso a datos, asegurando que las operaciones solicitadas se ejecuten de acuerdo con las reglas de negocio establecidas.
3. Capa de Acceso a Datos (Repository)
Esta capa interact煤a directamente con la fuente de datos (por ejemplo, una base de datos relacional) para realizar operaciones CRUD sobre las entidades de la aplicaci贸n: Producto, Cliente, y Venta. En Spring Boot, esto se logra mediante el uso de repositorios (anotados con @Repository
), que extienden interfaces como JpaRepository
para proporcionar m茅todos de acceso a datos listos para usar, as铆 como la capacidad de definir consultas personalizadas. Esta capa abstrae la complejidad del manejo directo de la base de datos de las otras capas de la aplicaci贸n.
4. Capa de Modelo (Model)
Finalmente, la capa de modelo define las entidades de negocio (Producto, Venta, Cliente) con sus respectivas propiedades y relaciones. Utilizando JPA (Java Persistence API) y anotaciones como @Entity
, Spring Boot mapea estas clases a tablas en la base de datos, facilitando la gesti贸n de la persistencia de datos y las relaciones entre entidades.
El proyecto aplica el modelo multicapas, separando las responsabilidades en distintas capas que interact煤an entre s铆 para facilitar el desarrollo, mantenimiento y escalabilidad de la aplicaci贸n. Spring Boot simplifica significativamente la implementaci贸n de cada capa mediante el uso de anotaciones y la inyecci贸n de dependencias, permitiendo a los desarrolladores concentrarse en la l贸gica de negocio y en proporcionar valor a los usuarios finales. Este enfoque asegura que la API pueda ser consumida tanto por aplicaciones web como m贸viles, cumpliendo con los requisitos de la due帽a de la tienda y adapt谩ndose a futuras expansiones o modificaciones.
En particular, en la imagen anterior se muestra la estructura de paquetes para la tienda descrita anteriormente. Esta estructura es un reflejo de la arquitectura en capas y la organizaci贸n de responsabilidades dentro del c贸digo.
La aplicaci贸n se divide en varios paquetes que agrupan clases e interfaces con responsabilidades similares:
Paquetes y su Rol en la Arquitectura Multicapas
-
com.marianadwarka.tienda.controller
- Contiene clases controladoras (
ClienteController
,ProductoController
,VentaController
) que act煤an como parte de la capa de presentaci贸n. Estas clases manejan las solicitudes HTTP entrantes, invocan operaciones de la capa de servicio y devuelven las respuestas adecuadas al cliente.
- Contiene clases controladoras (
-
com.marianadwarka.tienda.dto
- Define los objetos de transferencia de datos (DTOs, por sus siglas en ingl茅s
Data Transfer Objects
), comoMayorVentaDTO
yProductosPorVentaDTO
. Los DTOs se utilizan para transferir informaci贸n espec铆fica entre subcapas, especialmente de la l贸gica de negocio hacia la capa de presentaci贸n, facilitando el intercambio de datos y reduciendo la exposici贸n directa de las entidades del modelo.
- Define los objetos de transferencia de datos (DTOs, por sus siglas en ingl茅s
-
com.marianadwarka.tienda.model
- Contiene las entidades del dominio (
Cliente
,Producto
,Venta
) que representan la capa de modelo. Estas clases est谩n t铆picamente anotadas con@Entity
y son el reflejo de la estructura de la base de datos en c贸digo.
- Contiene las entidades del dominio (
-
com.marianadwarka.tienda.repository
- Alberga las interfaces para la capa de acceso a datos, como
IClienteRepository
,IProductoRepository
,IVentaRepository
. Estas interfaces extienden frameworks como JPA, permitiendo una abstracci贸n del acceso a la base de datos.
- Alberga las interfaces para la capa de acceso a datos, como
-
com.marianadwarka.tienda.service
- Incluye interfaces (
IClienteService
,IProductoService
,IVentaService
) y sus implementaciones (ClienteService
,ProductoService
,VentaService
). Las interfaces definen la l贸gica de negocio y las operaciones que pueden realizarse, mientras que las implementaciones concretan estas definiciones.
- Incluye interfaces (
Uso de Interfaces para las Capas de Servicio y Repositorio
Como se puede observar, se hizo la implementaci贸n de capas mediante interfaces, eesta es una pr谩ctica recomendada que tiene m煤ltiples ventajas, por ejemplo, las interfaces ayudan a desacoplar las capas de la aplicaci贸n. Por ejemplo, la capa de presentaci贸n no necesita saber c贸mo se implementan las operaciones en la capa de servicio, solo necesita conocer la interfaz. Esto facilita los cambios y la mantenibilidad del c贸digo.
Adem谩s, las interfaces permiten tener m煤ltiples implementaciones. Esto es 煤til si la l贸gica de negocio necesita variar seg煤n diferentes contextos, sin cambiar la forma en que los clientes de la capa de servicio interact煤an con ella.
Otro aspecto importante es que las interfaces act煤an como contratos que definen qu茅 operaciones est谩n disponibles, lo que ayuda a la claridad del dise帽o y a la expectativa de qu茅 hace cada parte del sistema.
La API desarrollada representa una soluci贸n t茅cnica para las necesidades operativas de una tienda en expansi贸n. Aprovechando las fortalezas de Spring Boot y la arquitectura multicapa, se ha logrado un sistema desacoplado que es f谩cil de probar, mantener y ampliar. La organizaci贸n del c贸digo en paquetes bien definidos, junto con la implementaci贸n de interfaces para las capas de servicio y acceso a datos, garantiza la capacidad de adaptar la aplicaci贸n a requisitos futuros con m铆nimo esfuerzo.